In JVM we have three layers,
they are:
1. Class
loader sub system
It is a part of JVM which
loads the class files into main memory of the computer.
While generating class files
by the JVM it checks for existence of java API which we are using
as a part of java program. It
the java API is not found it generates compilation error.
2. Runtime
data area:
When the class files are
loaded into main memory JVM requires the following
runtime data area, they are
Heap memory: It is used
for allocating the memory space for
at runtime.
Java
stacks/method area
amount of memory space in the
main memory on stack memory known as method area.
PC register: PC stands
for Program Counter which i
PC register always gives address of next instruction of java
program to the JVM.
JVM Architecture
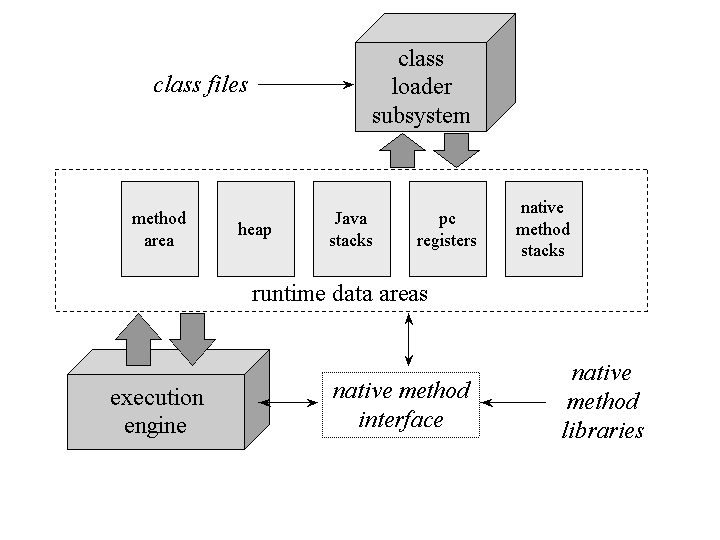
3. Execute
engine:
When a JVM is compiling in a
java program in that context JVM is known as
compiler. When a JVM is
running a java program in that context JVM is known as interpreter.
JVM reads the byte code of
instructions which are used
by JVM for generating the result of the java programs.
Method interface in th
JVM (execution engine) and java API. Java API contains
collection of packages.